Lecture 19 Accessibility
This chapter will discuss how to support Accessibility when developing Android applications—specifically, supporting users with levels of physical disability. Accessibility is an incredibly important software quality that is often overlooked, but making Android apps accessible requires only a few minor changes to the implementation details discussed in this course.
19.1 Universal Usability
When developing any kind of interactive system, there are different design principles (e.g., Shneiderman and Plaisant’s Golden Rules) that can provide guidelines for how to develop effective and usable system.
One of the most important design principles is Universal Usability (also known as Universal Design), which is the principle that designed products should be inherently accessible. This principle takes as its premise that designing for accessibility—to be usable by all people no matter their ability (physical or otherwise)—benefits not just those with some form of limitation or disability, but everyone.
The classic example of Universal Design are curb cuts: the “slopes” built into curbs to accommodate people in wheelchairs. However, this design decision end up making curbs more usability for everyone: curb cuts help people with rollerbags, strollers, temporary injuries, or who just have problems climbing steps.
- If you design a piece of technology to be used by a person with only one arm, then you support people with a disablity. But you also support people with a temporary disability (e.g., their arm is unusable because it is in a sling or a cast), and people who are just currently inconvenienced (e.g., they are holding a baby in that arm). You make the interaction and life better for everyone.
Universal usability is equally important in the domain of mobile design:
If you support people with vision impairments (e.g., by providing touch and voice controls), you also support people who just want to use the app while driving or otherwise visually occupied.
If you support people who cannot afford high-end devices with unlimited 4G connections (e.g., by functioning on older versions of Android, or being frugal when downloading data), you also support people who are currently without data connections (being out in the woords, on an airplane, over their data plan, etc).
People with disabilities cannot ethically be excluded from consideration in app design, and by considering their needs you will also improve the usability of your app for all population—two for the price of one! This guideline is increasingly being acknowledged by companies as key to usability, and thus it is important that you apply it to your own design work.
19.2 Implementing Accessibility
The Android framework provides a number of ways to make apps more accessible, including a handy accessibility developer checklist that you can follow. Some specific actions are described in more detail below: you should perform the listed tasks to test and improve an app’s accessibility.
These exercises build on the lecture code found at https://github.com/info448-s17/lecture07-loaders (use the completed
branch). You will need to adjust MainActivity
so that it shows the provided MovieFragment
by default; you will be testing and improving the accessibility of that Fragment.
19.2.1 Supporting Vision Impairment
One of the most important ways to support accessibility is to make sure your app is accessible to users with vision impairments.
As mentioned previously, one way to support users with trouble seeing content on small screens is to always use scalable pixels (sp
) as units on TextViews. This allows the size of the content to scale with user preferences: so if someone wants everything on the phone to be large, it can be!
However, users who are blind require extra support. This support is provided by Accessibility Services, which are “background services” that can respond to specific accessibility events (we will discuss Services more in a future lecture). The most common built-in service is called TalkBack, which is the Google-developed screen reader for Android. This service will “speak” the name of UI elements as the user focuses on them, as well as allow the user to drag a finger around a screen and get verbal feedback of what is there.
TalkBack can be turned enabled by going to
Settings > Accessibility > TalkBack
. This service is available on most consumer devices, but will need to be installed manually on the emulator. You can download the packaged.apk
from here (version 5.1.0 works fine), and install it on the emulator usingadb
on the Terminal:# replace with the package-name adb install package-name.apk
Turn on TalkBack and use it to explore your phone and the test the Loader Demo
app. You should do this without looking at your phone (avert you eyes, flip it upside down, etc)—try acting as if you were blind but still need to use the device!
The TalkBack service will start with a tutorial that you can complete (you can also read the user documentation).
In short: drag your finger to browse the device (letting TalkBack tell you what you are selecting), and then double-tap to “click” on an element.
As you should notice in testing your app, many interface designs give usability hints (e.g., what a button does) though visual cus: images, icons, and labels. While this may cause the app to “look” nice, it is not very effective for vision-impaired users—such as how the “icon” buttons are just explored as (e.g.) “Button 59”.
Thus for these purely visual elements (e.g., ImageButton
, ImageView
) we need to specify what text should be read by TalkBack. Do this by including an android:contentDescription
attribute on these elements, which are given a value of the text that TalkBack should read. Do this for all of the visual elements in the MovieFragment
layout. (You can also set this description for dynamic elements using the setContentDescription()
function in Java).
- This is equivalent to adding an
alt
or ARIA attribute in HTML.
Incuding the android:contentDescription
attribute is an incredibly easy addition (low-hanging fruit!) that does quite a lot to support accessibility of Android apps.
19.2.2 Supporting Alternative Inputs
A second easy change involves supporting interaction that doesn’t use the Touch Screen. This could be because of physical limitations: the user may interact with the device through an external device such as a keyboard, trackball, or switch.
The best way to support these alternative inputs is by making sure that each navigational element (things the user may select) are focusable. You can do this by specifying the android:focusable
attribute in the XML (or use the View#setFocusable()
method in Java).
Buttons are already focusable by default. But you can also specify the order by whch elements get focus (similar to the “tab order” in HTML). This is done using XML attributes android:nextFocusDown
, android:nextFocusUp
, android:nextFocusLeft
, android:nextFocusRight
. Each of these takes an id
reference as a value (e.g., "@id/nextElement"
), which refers to which View should gain focus instead of the “natural” order.
To practice this, modify the focus order so that the the “search input” has focus first, with the “search button” gaining focus on down from there and the “clear button” gaining focus on up.
In order to test this, you will need to make sure your device supports a physical keyboard and/or D-Pad, but you can also use the arrow keys for the emulator.
19.2.3 Supporting Internationalization
Finally you can make an application accessible to a wider diversity of users by providing Internationalization and supporting different languages and cultures.
Internationalization (i18n) is primarily done by specifying alternative resource, such as using XML to define user-facing Strings in multiple languages. We did an example of this in lecture 3
However, changes in language may also require adjustments to the layout resources themselves: phrases in some languages are significantly longer or shorter than in English, and so may cause problems with spacing or word wrapping.
- One way to test this is to enable pseudo-localization, a “fake” language that almost looks like English, but utilizes special characters and extraneous text to help test what the application may look like with different length content. See the link for details on enabling this.
Another significant change involves support right-to-left (RTL) languages such as Arabic. With these languages the “flow” of text goes in the opposite direction than in English, so many positioning elements in layouts may need to be reversed:
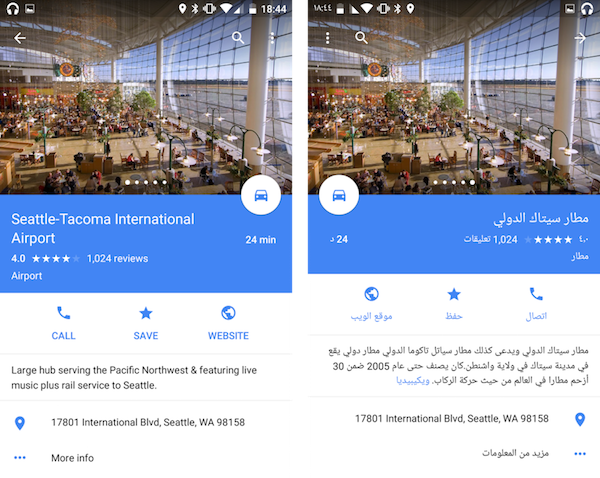
English and Arabic layouts. Image by Ryder Ziola.
In particular, do the following to best support both LTR and RTL languages:
Declare that your app supports RTL in the Manifest by including an attribute
android:supportsRtl="true"
in the<application>
element.With RelativeLayouts, use positioning attributes based on start and end rather than left and right. For example,
android:layout_toRightOf
should instead beandroid:layout_toEndOf
. This will allow the relative positioning to automatically “switch” between LTR and RTL. Note that LinearLayouts automatically reverse direction!For custom icons and drawables: define separate resources for LTR and RTL (use the
ldrtl
resource qualitifier to specify the “layout direction” as right-to-left). This will allow for icons (such as the arrows in the upper start corner of the example) to change direction with the text—you want “back” to actually point “back”!
Make these changes to the MovieFragment
(there are no custom drawables to adjust). You can test that your changes work by selecting Settings > Developer options > Force RTL layout direction
.
19.2.4 Further Testing
These are fairly trivial changes you can make to how you define and implement user interfaces, that will go a long way to supporting use by all users no matter their level of ability.
The Android Studio IDE will identify and suggest additional changes as “linted” style suggestions—watch out for these warnings and learn to correct them as you develop.
Finally, Google has also developed an Accessibility Scanner app that can be used to check for further accessibility issues (such as contrast levels or touchable areas).
And of course, keep the checklist handy to help you design applications to support universal usability.